Introduction
The C programming language is a general purpose programming language, which relates closely to the way machines work. Understanding how computer memory works is an important aspect of the C programming language. Although C can be considered as "hard to learn", C is in fact a very simple language, with very powerful capabilities.
C is a very common language, and it is the language of many applications such as Windows, the Python interpreter, Git, and many many more.
C is a compiled language - which means that in order to run it, the compiler (for example, GCC or Visual Studio) must take the code that we wrote, process it, and then create an executable file. This file can then be executed, and will do what we intended for the program to do.
C is a general-purpose programming language used for wide range of applications from Operating systems like Windows and iOS to software that is used for creating 3D movies.
C programming is highly efficient. That’s the main reason why it’s very popular despite being more than 40 years old.
Standard C programs are portable. The source code written in one system works in another operating system without any change.
As mentioned, it’s a good language to start learning programming. If you know C programming, you will not just understand how your program works, but will also be able to create a mental picture on how a computer works.
Compile and run C programming on your OS
There are numerous compilers and text editors you can use to run C programming. These compilers and text editors may differ from system to system.
You will find the easiest way to run C programming on your computer (Windows, Mac OS X or Linux) in this section.
- There are several sites that allows you to run C programming online. The one I prefer is ideone.com.
- To run C programming in ideone.com, select C language at the bottom of the editor, write C code and click Run.
- It should be noted that, online C compilers are limited (you can’t work with files, taking input from the user is not natural and so on).
Run C Programming in Mac OS X :
- Go to download page of apple developer site.
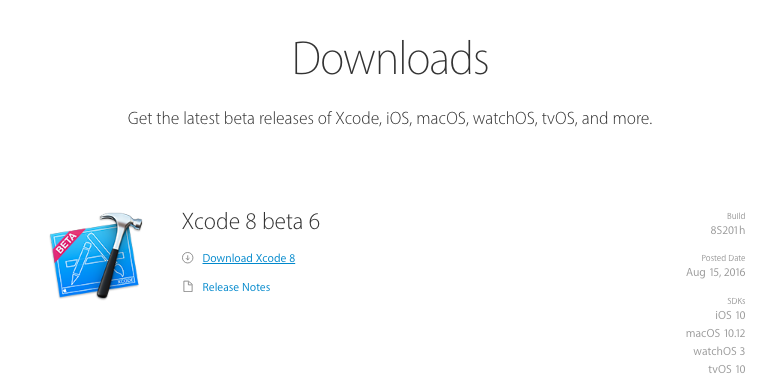
- Click the download Xcode link. It’s recommended to download the latest version even if it’s in beta.
- When download is completed, open Xcode and follow the wizard to install it. You might want to put the Xcode in Applications for future use.
- Then, open Xcode and go to File > New > Project.
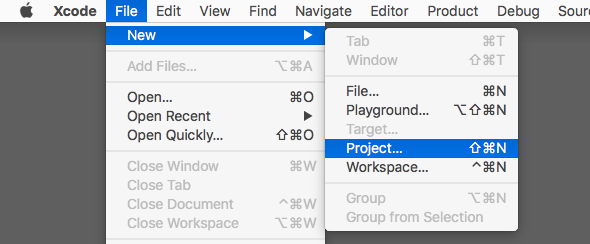
- Under OS X section, choose Application, choose Command Line Tool and hit next.
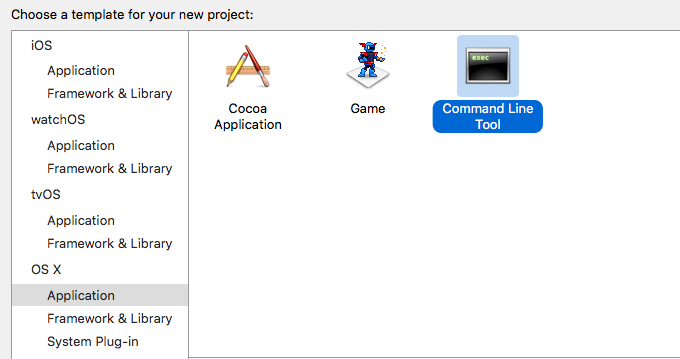
- Provide the Product Name, for example: Hello. And, choose C under Language section. Then, click next.
- Choose the location where you want to save the project in your Mac. You can uncheck Create Git repository button and click create.
- Navigate to main.c file in the screen where you can see default code provided by XCode.
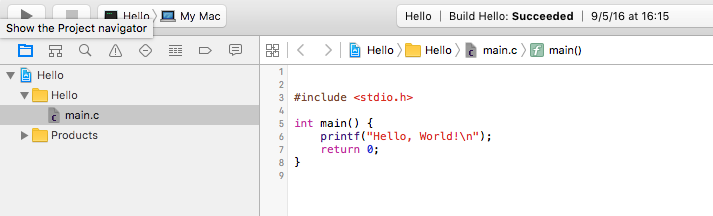
- Change the code as you wish. And finally to run the code, go to Product > Run or simply hit Cmd + R.
- By default you will see the output at the bottom of your screen.
Run C programming on Linux:
To run C programming on Linux, you need:
- A compiler. We’ll install GNU GCC compiler which is good for beginners.
- Development tools and libraries.
- A text editor (gEdit works just fine for our purpose). Or, you can download text editor of your choice.
- Open the terminal and write the following command.
For Ubuntu and Debian distribution:
$ sudo apt-get update
$ sudo apt-get install build-essential manpages-dev
For Cent OS, Fedora, Red Hat and Scientific Linux:
# yum groupinstall 'Development Tools'
This installs GNU GCC compiler and related tools on your system.
This installs GNU GCC compiler and related tools on your system.
- To verify if GCC compiler is installed, issue the command.
$ gcc --version
If you get output similar like this, gcc is correctly installed on your system.
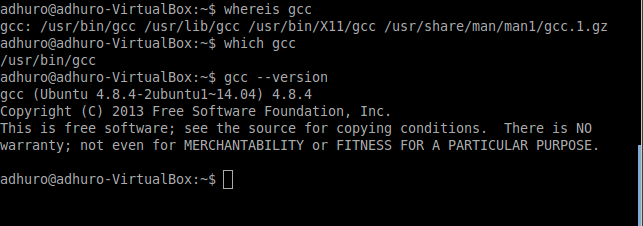
- Open the text editor of your choice and save a file with .c extension. I made hello.c file using gEdit.
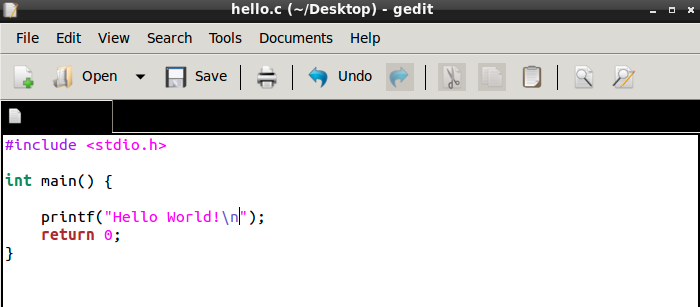
If you are a Linux wizard, feel free to use vim or emacs. Any editor is fine but, don’t forget to use .c extension; it’s important.
- Switch to the directory where the file is located. And, issue the following command.
$ gcc program-source-code.c -o name-of-your-choice
Here, program-source.code.c is the filename you chose before. And, name-of-your-choice can be any name you prefer. In my case, I issued the following command. $ gcc hello.c -o hello.
- If there is no error, an executable file named is created; hello is my case.
- Finally, you can see the output using following command.
$ ./hello
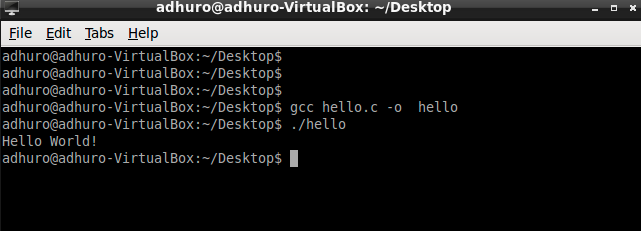
As you might have imagined, you need to use the name of executable file you chose before. Also, you need to use path to the execute file if you are in a different directory.
Run C Programming in Windows (XP, 7, 8 and 10) :
To make this procedure even easier, follow this step by step guide.
- Go to the binary release download page of Code:Blocks official site.
- Under Windows XP / Vista / 7 / 8.x / 10 section, click the link with mingw-setup (highlighted row) either from Sourceforge.net or FossHub.
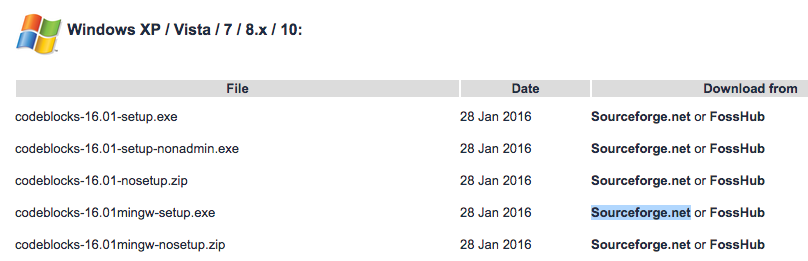
- Open the Code::Blocks Setup file and follow the instructions (Next > I agree > Next > Install); you don’t need to change anything. This installs the Code::Blocks with gnu gcc compiler, which is the best compiler to start with for beginners.
- Now, open Code::Blocks and go to File > New > Empty file (Shortcut: Ctrl + Shift + N)
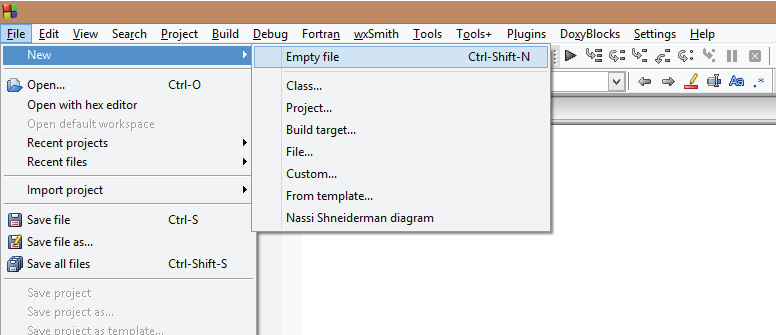
- Write the C code and save the file with .c extension. To save the file, go to File > Save (Shortcut: Ctrl + S). Important: The filename should end with a .c extension, like: hello.c, your-program-name.c
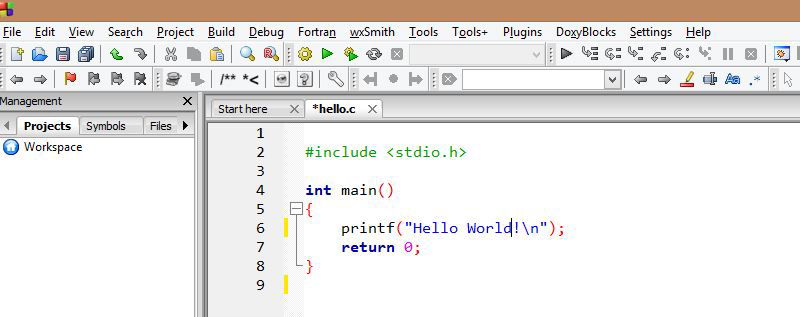
- To run the program, go to Build > Build and Run (Shortcut: F9). This will build the executable file and run it.
If your program doesn’t run and if you see error message "can't find compiler executable in your search path(GNU GCC compiler)", go to Settings > Compiler > Toolchain executables and click Auto-detect. This should solve the issue in most cases.
C Programs
A C program can vary from 3 lines to millions of lines and it should be written into one or more text files with extension ".c"; for example, hello.c. You can use "vi", "vim" or any other text editor to write your C program into a file.
This tutorial assumes that you know how to edit a text file and how to write source code inside a program file.
Your First Program
You will learn to write a “Hello, World!” program in this section.
Before we study the basic building blocks of the C programming language, let us look at a bare minimum C program structure so that we can take it as a reference in the upcoming chapters.
A C program basically consists of the following parts :
- Preprocessor Commands
- Functions
- Variables
- Statements & Expressions
- Comments
Let us look at a simple code that would print the words "Hello World" :
#include <stdio.h>
int main()
{
/* my first program in C */
printf("Hello World! \n");
return 0;
}
int main()
{
/* my first program in C */
printf("Hello World! \n");
return 0;
}
How The Above Program Works?
$ gcc hello.c
- C programming is small and cannot do much by itself. You need to use libraries that are necessary to run the program. The stdio.h is a header file and C compiler knows the location of that file. The first line of the program #include <stdio.h> is a preprocessor command, which tells a C compiler to include stdio.h file before going to actual compilation.
- In C programming, the code execution begins from the start of main() function (doesn’t matter if main() isn’t located at the beginning). The code inside the curly braces { } is the body of main() function. The main() function is mandatory in every C program.
- The next line /*...*/ will be ignored by the compiler and it has been put to add additional comments in the program. So such lines are called comments in the program.
- The printf( ) Function is a library function that sends formatted output to the screen (displays the string inside the quotation mark). Notice the semicolon at the end of the statement. In our program, it displays Hello World! on the screen. Remember, you need to include stdio.h file in your program for this to work.
- The return statement return 0; inside the main() function ends the program. This statement isn’t mandatory. However, it’s considered good programming practice to use it.
How Do I Save And Run This Program?
Let us see how to save the source code in a file, and how to compile and run it. Following are the simple steps −
- Open a text editor and add the above-mentioned code.
- Save the file as hello.c
- Open a command prompt and go to the directory where you have saved the file.
- Type gcc hello.c and press enter to compile your code.
- If there are no errors in your code, the command prompt will take you to the next line and would generate a.out executable file.
- Now, type a.out to execute your program.
$ gcc hello.c
$ ./a.out
Hello, World!
Make sure the gcc compiler is in your path and that you are running it in the directory containing the source file hello.c.
Tips To Remember:
- All C program starts from the main() function and it’s mandatory.
- You can use the required header file that’s necessary in the program. For example: To use sqrt() function to calculate square root and pow() function to find power of a number, you need to include math.h header file in your program.
- C is case-sensitive; the use of uppercase letter and lowercase letter have different meanings.
- The C program ends when the program encounters the return statement inside the main() function. However, return statement inside the main function is not mandatory.
- The statement in a C program ends with a semicolon.
Reference:
Note:
I Don't have any copyrights to the content in this post.The content doesn't belongs to me. I have just merged important contents of more than one site to provide with a rich content to learn easily.
No comments:
Post a Comment